How To Check If A Set Is Empty In Python
A gear up is an unordered collection of items. Every set element is unique (no duplicates) and must be immutable (cannot be inverse).
However, a set itself is mutable. We can add or remove items from it.
Sets can also be used to perform mathematical set operations like marriage, intersection, symmetric difference, etc.
Creating Python Sets
A set is created by placing all the items (elements) within curly braces {}
, separated by comma, or by using the built-in gear up()
function.
It tin have whatsoever number of items and they may be of dissimilar types (integer, float, tuple, string etc.). But a fix cannot take mutable elements like lists, sets or dictionaries as its elements.
# Unlike types of sets in Python # gear up of integers my_set = {1, 2, iii} impress(my_set) # set of mixed datatypes my_set = {one.0, "Hello", (i, 2, 3)} print(my_set)
Output
{1, 2, 3} {1.0, (1, ii, 3), 'Hullo'}
Try the post-obit examples likewise.
# set cannot have duplicates # Output: {1, 2, 3, 4} my_set = {i, 2, 3, four, iii, 2} print(my_set) # we can brand prepare from a listing # Output: {1, ii, 3} my_set = set([1, 2, three, 2]) print(my_set) # ready cannot have mutable items # here [3, 4] is a mutable list # this will cause an error. my_set = {one, two, [3, four]}
Output
{1, 2, 3, iv} {1, two, iii} Traceback (most recent call final): File "<string>", line 15, in <module> my_set = {1, 2, [iii, 4]} TypeError: unhashable blazon: 'list'
Creating an empty fix is a bit catchy.
Empty curly braces {}
will make an empty dictionary in Python. To make a fix without any elements, we employ the set()
function without any argument.
# Distinguish set and dictionary while creating empty set # initialize a with {} a = {} # bank check data type of a impress(type(a)) # initialize a with set() a = set() # check data type of a print(type(a))
Output
<class 'dict'> <grade 'set'>
Modifying a gear up in Python
Sets are mutable. Yet, since they are unordered, indexing has no meaning.
We cannot access or change an element of a set using indexing or slicing. Set up information type does not support it.
We can add together a unmarried element using the add()
method, and multiple elements using the update()
method. The update()
method tin can take tuples, lists, strings or other sets as its statement. In all cases, duplicates are avoided.
# initialize my_set my_set = {one, 3} print(my_set) # my_set[0] # if you lot uncomment the above line # you volition get an fault # TypeError: 'set' object does not support indexing # add an chemical element # Output: {1, 2, 3} my_set.add(ii) print(my_set) # add together multiple elements # Output: {ane, ii, 3, 4} my_set.update([2, three, 4]) print(my_set) # add together list and ready # Output: {ane, 2, iii, 4, 5, 6, 8} my_set.update([4, 5], {one, 6, viii}) print(my_set)
Output
{1, 3} {1, 2, iii} {ane, 2, 3, four} {1, 2, 3, 4, 5, 6, 8}
Removing elements from a set
A particular item tin be removed from a set using the methods discard()
and remove()
.
The but difference betwixt the two is that the discard()
function leaves a set unchanged if the element is not present in the ready. On the other hand, the remove()
function will enhance an mistake in such a status (if element is not present in the set).
The following case will illustrate this.
# Difference betwixt discard() and remove() # initialize my_set my_set = {ane, 3, iv, 5, half-dozen} print(my_set) # discard an element # Output: {i, three, 5, six} my_set.discard(four) print(my_set) # remove an element # Output: {1, 3, v} my_set.remove(6) print(my_set) # discard an element # not present in my_set # Output: {1, 3, five} my_set.discard(2) print(my_set) # remove an element # not nowadays in my_set # you lot will become an mistake. # Output: KeyError my_set.remove(2)
Output
{1, 3, 4, five, six} {1, 3, 5, 6} {1, three, 5} {one, 3, v} Traceback (almost recent call concluding): File "<string>", line 28, in <module> KeyError: 2
Similarly, nosotros tin remove and return an detail using the pop()
method.
Since prepare is an unordered information blazon, there is no manner of determining which detail will be popped. Information technology is completely arbitrary.
We tin besides remove all the items from a set using the clear()
method.
# initialize my_set # Output: set of unique elements my_set = set("HelloWorld") impress(my_set) # pop an element # Output: random element print(my_set.pop()) # pop another element my_set.pop() print(my_set) # clear my_set # Output: set() my_set.articulate() impress(my_set)
Output
{'H', 'l', 'r', 'W', 'o', 'd', 'due east'} H {'r', 'W', 'o', 'd', 'eastward'} set()
Python Set Operations
Sets tin be used to behave out mathematical prepare operations like union, intersection, departure and symmetric difference. We tin do this with operators or methods.
Let united states consider the following two sets for the following operations.
>>> A = {1, two, 3, iv, v} >>> B = {4, five, half dozen, 7, 8}
Set Union
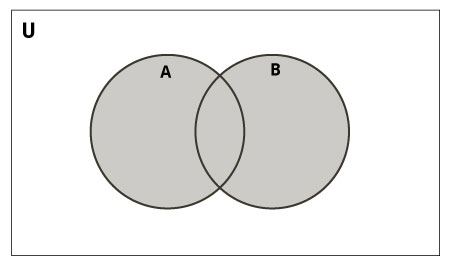
Union of A and B is a set of all elements from both sets.
Union is performed using |
operator. Same can be accomplished using the wedlock()
method.
# Set spousal relationship method # initialize A and B A = {one, 2, 3, iv, 5} B = {iv, 5, 6, 7, 8} # use | operator # Output: {1, 2, 3, 4, 5, six, vii, 8} impress(A | B)
Output
{1, 2, 3, iv, 5, 6, seven, 8}
Endeavor the following examples on Python beat.
# utilise marriage function >>> A.union(B) {one, 2, 3, 4, 5, half dozen, 7, eight} # use union function on B >>> B.marriage(A) {1, ii, 3, 4, 5, 6, vii, 8}
Set Intersection
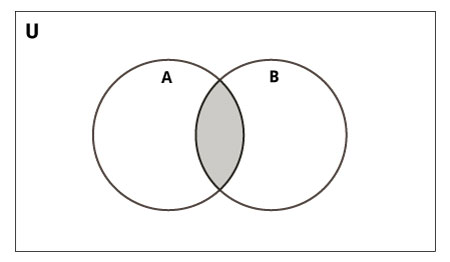
Intersection of A and B is a set of elements that are common in both the sets.
Intersection is performed using &
operator. Same can be accomplished using the intersection()
method.
# Intersection of sets # initialize A and B A = {one, 2, three, four, five} B = {four, 5, half-dozen, vii, 8} # apply & operator # Output: {4, 5} print(A & B)
Output
{4, five}
Try the following examples on Python beat.
# employ intersection function on A >>> A.intersection(B) {4, v} # use intersection role on B >>> B.intersection(A) {four, 5}
Prepare Difference
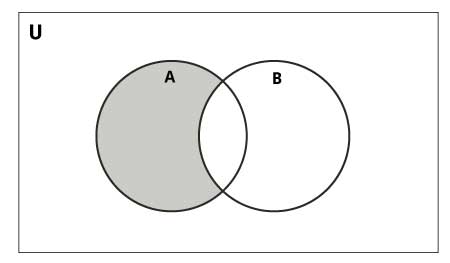
Difference of the set B from set A(A - B) is a set of elements that are but in A but non in B. Similarly, B - A is a prepare of elements in B simply not in A.
Difference is performed using -
operator. Aforementioned can be accomplished using the deviation()
method.
# Difference of 2 sets # initialize A and B A = {one, two, iii, iv, 5} B = {iv, v, half-dozen, 7, 8} # use - operator on A # Output: {1, 2, 3} print(A - B)
Output
{1, ii, 3}
Try the following examples on Python crush.
# utilise departure office on A >>> A.deviation(B) {1, two, iii} # use - operator on B >>> B - A {viii, 6, seven} # employ difference function on B >>> B.departure(A) {eight, 6, 7}
Prepare Symmetric Departure
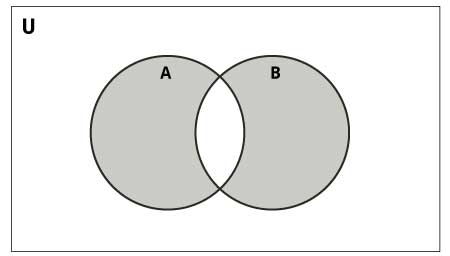
Symmetric Difference of A and B is a set up of elements in A and B simply not in both (excluding the intersection).
Symmetric difference is performed using ^
operator. Same can be accomplished using the method symmetric_difference()
.
# Symmetric difference of two sets # initialize A and B A = {1, two, 3, 4, 5} B = {4, five, 6, 7, eight} # use ^ operator # Output: {1, ii, three, six, 7, viii} print(A ^ B)
Output
{one, 2, 3, six, 7, 8}
Try the following examples on Python shell.
# use symmetric_difference role on A >>> A.symmetric_difference(B) {i, 2, three, 6, 7, 8} # use symmetric_difference part on B >>> B.symmetric_difference(A) {one, 2, 3, vi, 7, eight}
Other Python Ready Methods
There are many set methods, some of which we have already used in a higher place. Hither is a list of all the methods that are bachelor with the prepare objects:
Method | Description |
---|---|
add() | Adds an element to the fix |
articulate() | Removes all elements from the prepare |
copy() | Returns a copy of the fix |
divergence() | Returns the difference of two or more sets equally a new set |
difference_update() | Removes all elements of some other set from this prepare |
discard() | Removes an element from the set up if information technology is a member. (Practise nothing if the chemical element is not in set) |
intersection() | Returns the intersection of 2 sets as a new set |
intersection_update() | Updates the set with the intersection of itself and another |
isdisjoint() | Returns Truthful if two sets have a zero intersection |
issubset() | Returns Truthful if some other set up contains this set |
issuperset() | Returns Truthful if this set contains another set |
pop() | Removes and returns an capricious set element. Raises KeyError if the set is empty |
remove() | Removes an chemical element from the prepare. If the element is non a fellow member, raises a KeyError |
symmetric_difference() | Returns the symmetric departure of two sets equally a new ready |
symmetric_difference_update() | Updates a set with the symmetric deviation of itself and another |
union() | Returns the wedlock of sets in a new set |
update() | Updates the set with the spousal relationship of itself and others |
Other Set Operations
Set Membership Examination
We can test if an item exists in a ready or not, using the in
keyword.
# in keyword in a set up # initialize my_set my_set = prepare("apple") # check if 'a' is present # Output: True print('a' in my_set) # cheque if 'p' is present # Output: False print('p' non in my_set)
Output
True False
Iterating Through a Set
We tin iterate through each particular in a set using a for
loop.
>>> for letter of the alphabet in set("apple"): ... print(alphabetic character) ... a p e 50
Congenital-in Functions with Set up
Built-in functions like all()
, any()
, enumerate()
, len()
, max()
, min()
, sorted()
, sum()
etc. are unremarkably used with sets to perform different tasks.
Part | Description |
---|---|
all() | Returns True if all elements of the set are true (or if the set is empty). |
any() | Returns True if any element of the ready is true. If the set is empty, returns Fake . |
enumerate() | Returns an enumerate object. Information technology contains the index and value for all the items of the ready as a pair. |
len() | Returns the length (the number of items) in the prepare. |
max() | Returns the largest item in the fix. |
min() | Returns the smallest item in the set. |
sorted() | Returns a new sorted list from elements in the set up(does not sort the set up itself). |
sum() | Returns the sum of all elements in the set up. |
Python Frozenset
Frozenset is a new class that has the characteristics of a set, merely its elements cannot be changed once assigned. While tuples are immutable lists, frozensets are immutable sets.
Sets being mutable are unhashable, and so they can't be used as lexicon keys. On the other manus, frozensets are hashable and tin be used as keys to a dictionary.
Frozensets can be created using the frozenset() office.
This information type supports methods similar copy()
, difference()
, intersection()
, isdisjoint()
, issubset()
, issuperset()
, symmetric_difference()
and union()
. Existence immutable, it does not have methods that add or remove elements.
# Frozensets # initialize A and B A = frozenset([1, two, 3, iv]) B = frozenset([3, four, 5, half-dozen])
Attempt these examples on Python beat out.
>>> A.isdisjoint(B) False >>> A.divergence(B) frozenset({1, 2}) >>> A | B frozenset({1, two, iii, 4, 5, 6}) >>> A.add together(three) ... AttributeError: 'frozenset' object has no attribute 'add'
Source: https://www.programiz.com/python-programming/set
0 Response to "How To Check If A Set Is Empty In Python"
Post a Comment